DLL Operation: Delete
- Trace the basic operations of a (doubly) linked-list implementation.
- Understand the basic operations of a (doubly) linked list well enough to implement them.
Suppose we want to delete the node pointed to by the reference variable target
:

Exercise Complete the implementation of delete
.
public void delete(Node<T> target) {
// TODO Implement me!
}
Hint: Use the following visualization as guidance.
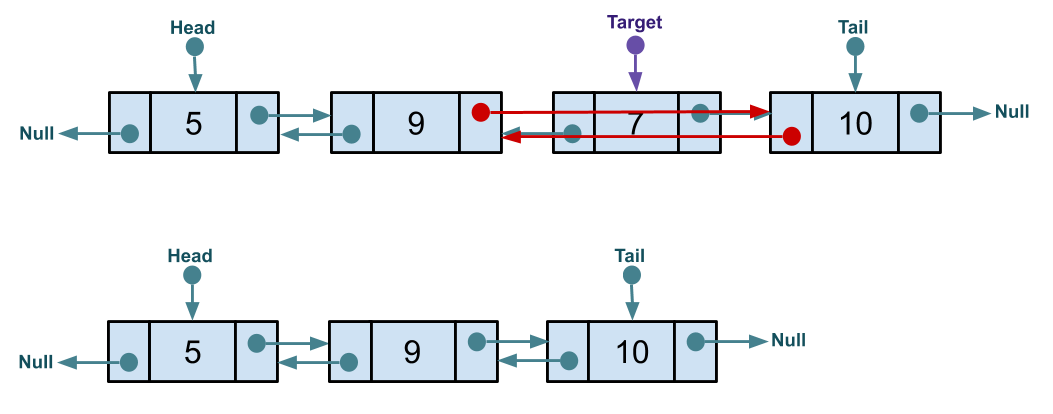
Solution
public void delete(Node<T> target) {
Node<T> prevNode = target.prev;
Node<T> nextNode = target.next;
prevNode.next = nextNode;
nextNode.prev = prevNode;
// no need for these!
target.next = null;
target.prev = null;
}
Caution: the implementation above fails to account for edge cases!