Class Diagram
- Catalogue class relationships into is-a and has-a classes and further separate is-a relationships into "extends" and "implements" types.
We have created a simple shape drawing application that can compose basic geometric shapes into more complex ones, such as the smiley face below!
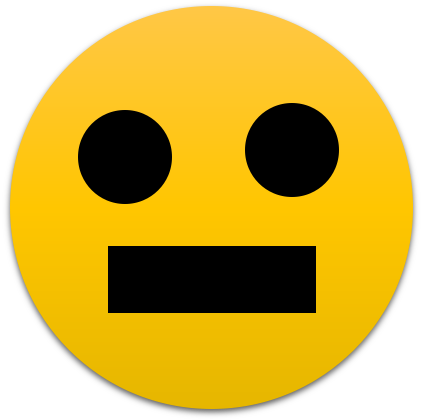
Here is a partial class diagram of our software. The links between classes are removed!
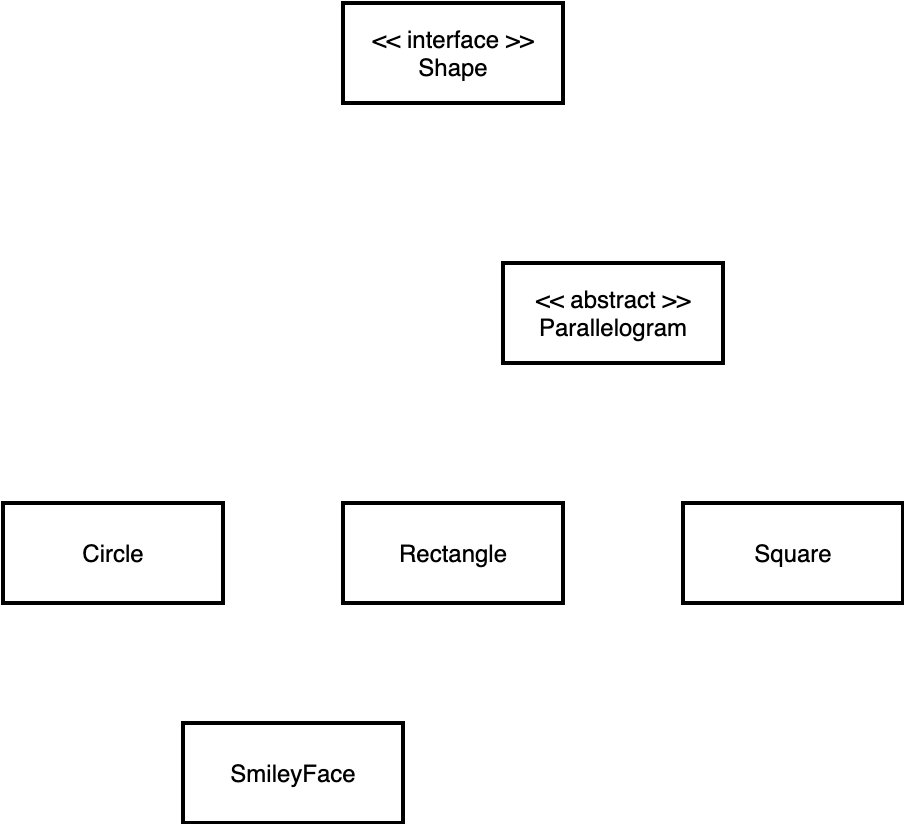
Exercise Add links (arrows) between classes to indicate "is-a" and "has-a" relationship. Catalogue is-a relationships into "extends" and "implements" categories.
Solution
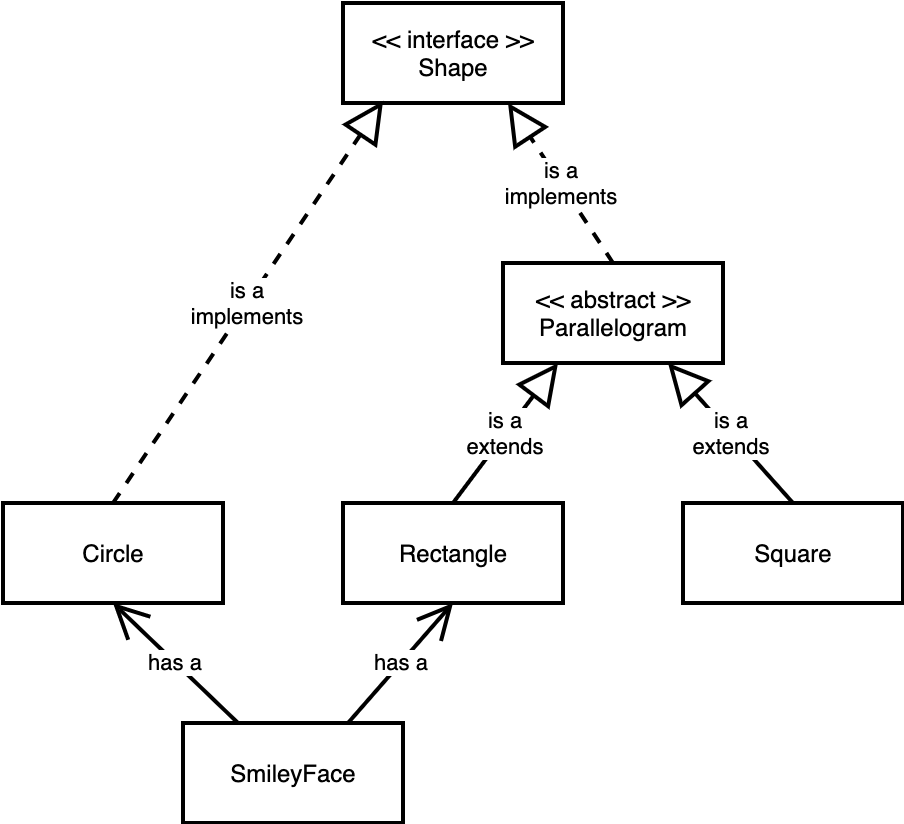
There could be an "is-a" link between SmileyFace
and Shape
too.
Exercise Based on the completed diagram, make up an example to showcase polymorphism. (Do this at home!)
Solution
Imagine there is an area
method in the Shape
interface. This method must be implemented appropriately in the Circle
and Square
classes.
Further, assume there is the following method:
public static void printArea(Shape s) {
System.out.println(s.area());
}
The following code exhibits polymorphism:
Circle ci = new Circle(2);
Square sq = new Square(3);
printArea(ci);
printArea(sq);
-
Static polymorphism: During compile-time,
ci
andsq
are implicitly upcasted toShape
whenprintArea
is called. -
Dynamic polymorphism: During runtime, JVM dispatches the correct implementation of
s.area()
based on the actual type ofs
(which is, for example,Circle
whenci
is passed toprintArea
).