DLL Operation: Prepend
- Trace the basic operations of a (doubly) linked-list implementation.
- Understand the basic operations of a (doubly) linked list well enough to implement them.
Suppose we have the following DLL, and we want to add a new node to its front.

Exercise Complete the implementation of the addFirst
method that creates a node and adds it to the front of the list.
public void addFirst (T data) {
// TODO Implement Me!
}
Hint: Use the following visualization as a guidance:
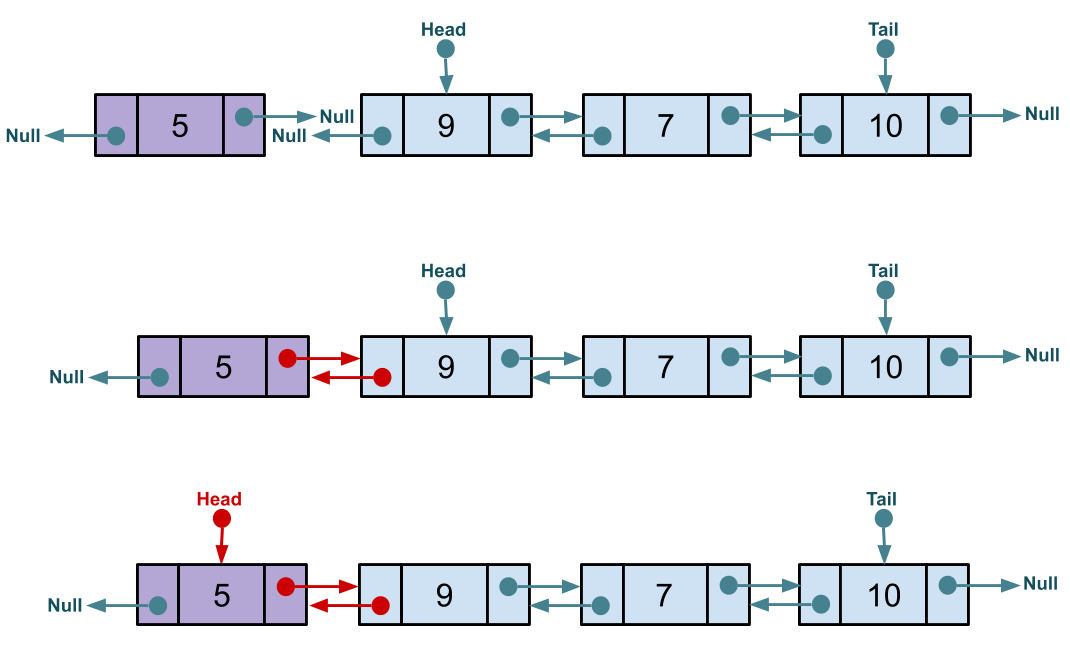
Solution
public void addFirst(T data) {
Node<T> node = new Node<>(data);
node.next = head;
head.prev = node;
head = node;
}
Caution: the implementation above fails to account for edge cases!