Two Types of Exceptions
- Distinguish checked vs. unchecked exceptions.
There are two kinds of exceptions in Java: checked exceptions and unchecked exceptions. In this lesson, I'll provide some examples to understand the distinction.
Checked Exceptions
Consider this code:
public void doSomething() {
doThisFirst();
// more code here!
}
If doThisFirst
method throws a checked exception, the compiler will not let you simply call it! You must handle the exception by either of these techniques:
- Using
try
/catch
block
public void doSomething() {
try {
doThisFirst();
} catch (SomeCheckedException e) {
// Deal with the exception.
}
// more code here!
}
- Allow the
doSomething
method to throw the exception raised bydoThisFirst
public void doSomething() throws SomeCheckedException {
doThisFirst();
// more code here!
}
A method that throws a checked exception must include a throws
clause in its declaration.
Java verifies checked exceptions at compile-time and forces you to provide a handling mechanism for it.
Unchecked Exceptions
All Java exceptions are checked unless they subtype the Error
class or the RuntimeException
class.
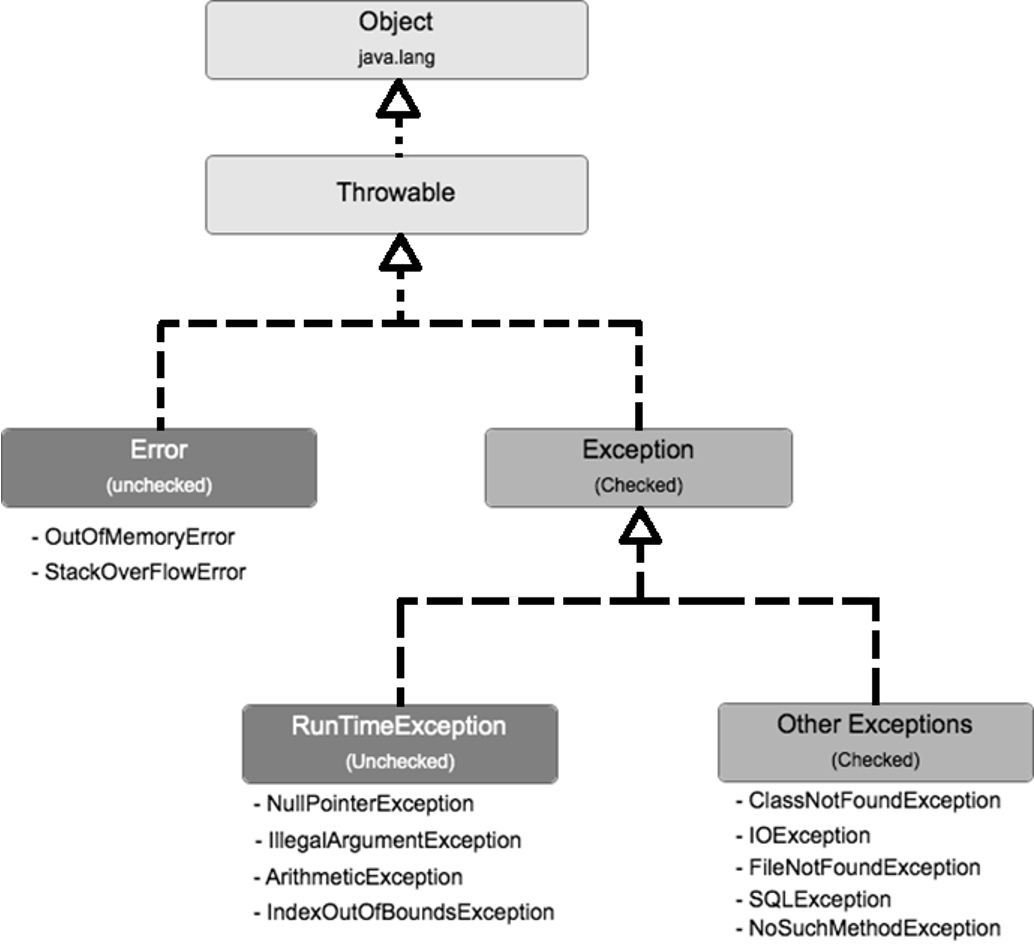
The compiler does not force you to handle unchecked exceptions. For instance, the compiler will let you simply call the doThisFirst()
if it (may potentially) throws an unchecked exception:
public void doSomething() {
doThisFirst();
// more code here!
}
A method that throws an unchecked exception does not need to include a throws
clause in its declaration.
Why two types of Exceptions?
The distinction between checked v.s. unchecked exception is specific to Java. In C++
, for instance, all exceptions are unchecked, so it is up to the programmer to handle them or not.
Read Unchecked Exceptions — The Controversy on Oracle's website for more information. In a nutshell, Java believes it must force programmers to handle exceptions. But, on the other hand, it makes allowance to declare errors that could happen, but rarely do, as unchecked exceptions. So, the programmer is not frustrated to, e.g., have to write try/catch every time they use division in a program even though division by zero causes ArithmeticException
.
Resources
Checked and Unchecked Exceptions in Java by Baeldung is a good read.