DLL Operation: Append
- Trace the basic operations of a (doubly) linked-list implementation.
- Understand the basic operations of a (doubly) linked list well enough to implement them.
Suppose we have the following DLL, and we want to append (add to the end) a new node.

Exercise Complete the implementation of the addLast
method that creates a node and add it to the back of the list.
public void addLast(T data) {
// TODO Implement me!
}
Hint: Use the following visualization as guidance.
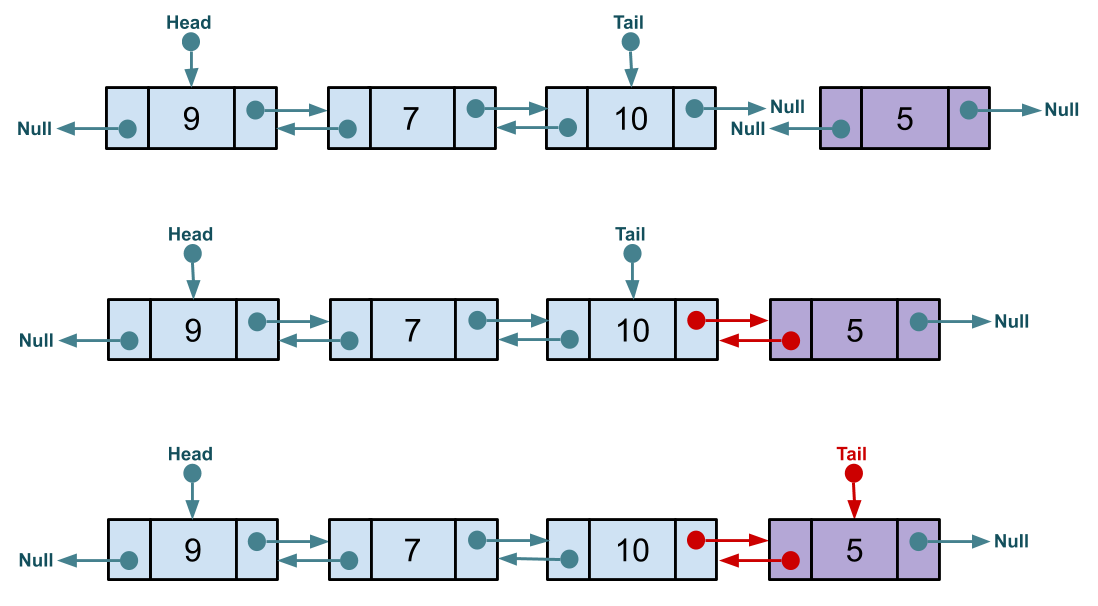
Solution
public void addLast(T data) {
Node<T> node = new Node<>(data);
node.prev = tail;
tail.next = node;
tail = node;
}
Caution: the implementation above fails to account for edge cases!