Use Descriptive Names!
- Express best practices of unit testing.
Here is our unit test again:
@Test
void length() {
IndexedList<Integer> numbers = new ArrayIndexedList<>(5, 10);
assertEquals(5, numbers.length());
}
I prefer to rename the test so that it better reflects what is being tested.
@Test
void testLengthAfterConstruction() {
IndexedList<Integer> numbers = new ArrayIndexedList<>(5, 10);
assertEquals(5, numbers.length());
}
We can optionally use the @DisplayName
annotation to provide a more elaborate description.
@Test
@DisplayName("length() returns the correct size after IndexedList is instantiated.")
void testLengthAfterConstruction() {
IndexedList<Integer> numbers = new ArrayIndexedList<>(5, 10);
assertEquals(5, numbers.length());
}
When you run the test, you must see the following in IntelliJ
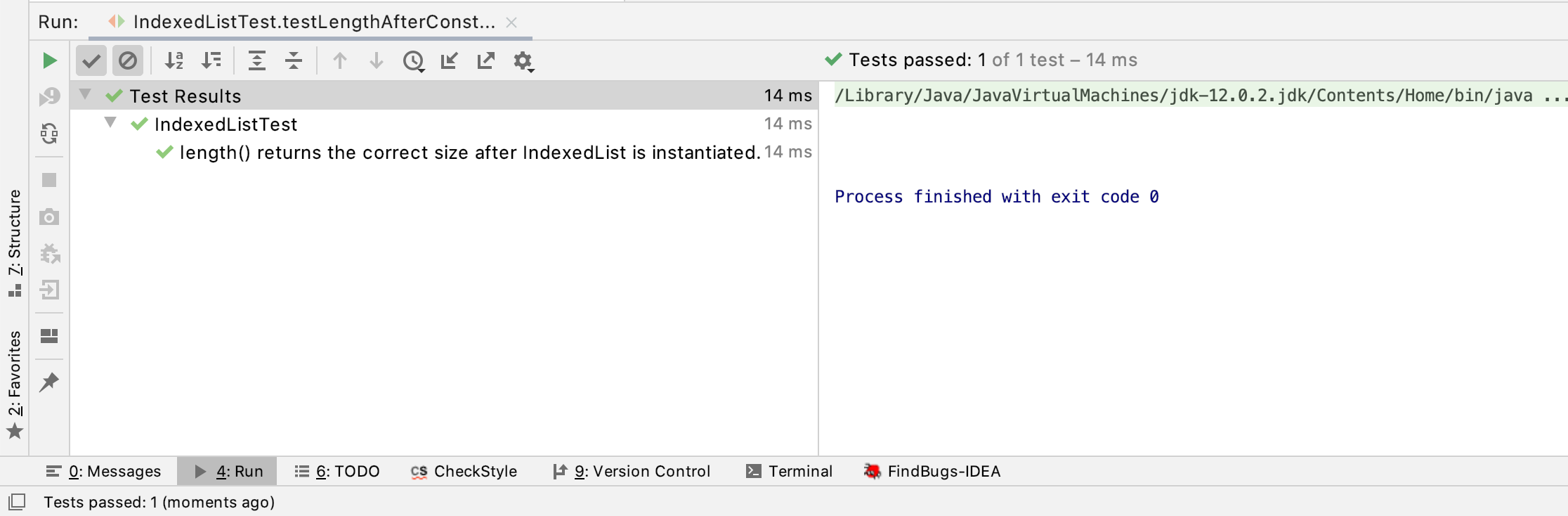
You are not required to use @DisplayName
annotations. However, your unit tests must be named descriptively. Never, ever, name them generically as test1
, test2
, etc.