JUnit Framework
- Apply the JUnit framework to unit testing Java code.
- Define what a unit test is.
In this course, we use a Java library called JUnit to write and run tests.
JUnit provides annotations to write tests using the familiar structure and syntax of Java classes and methods but treats them as "tests" once annotated as one.
The library is included in the lib
folder, which we provide as the starter/solution for homework and chapters.
Let's create a test class for the IndexedList ADT:
import org.junit.jupiter.api.Test;
class IndexedListTest {
@Test
void put() {
}
@Test
void get() {
}
@Test
void length() {
}
}
You must store the IndexedListTest.java
file inside the folder src/test/java
.
Conventionally, a test class has the word "Test" in its name, either preceding or following the class name the tests are written for.
Each method that is annotated with @Test
is a unit test.
A "unit test" is a term used in software engineering to refer to a test written to examine the smallest block of code that can be isolated for testing. In most programming languages, such a "unit" is a function/method.
Notice, to use @Test
annotation; to use it, you must have the following import at the top of your source code.
import org.junit.jupiter.api.Test;
IntelliJ is equipped with a plugin that facilitates running JUnit tests. For example, you can click on the green play arrow next to each test to run it.
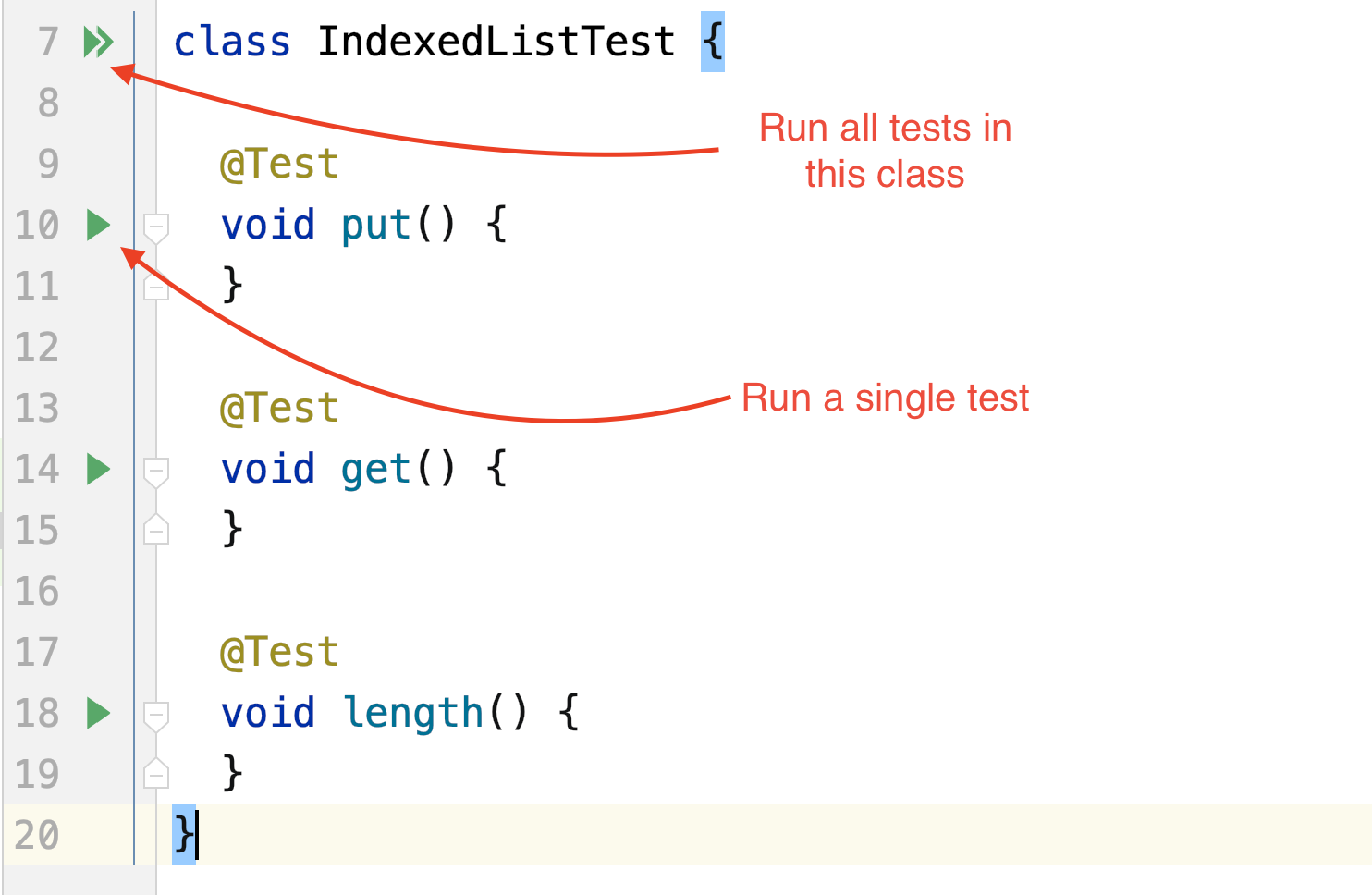
Resources
- There is a detailed User Guide on JUnit's website.
- Baeldung has several articles on using JUnit, from testing fundamental to advanced topics.