Traversing a Linked List
- Trace the basic operations of a (singly) linked-list implementation.
- Understand the basic operations of a (singly) linked list well enough to implement them.
Suppose we have a linked list with $n$ elements (nodes), and we want to go over every element and print out the data stored in it.
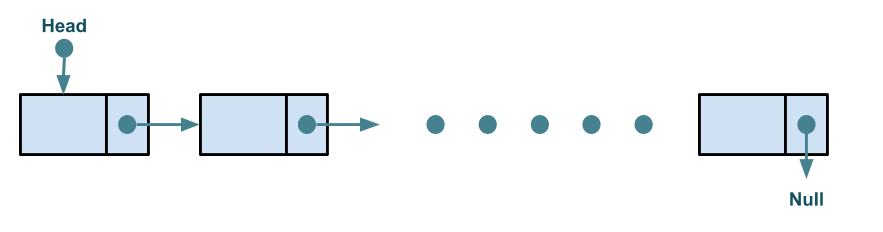
Exercise Complete the implementation of traverse
that iterates over a linked list and prints the data stored at every node.
public void traverse() {
// TODO Implement me!
}
Hint: the front of the linked list is marked by the head
pointer. If you were to follow the next
references, how would you know when you reached the last node?
Solution
public void traverse() {
Node<T> current = head;
while (current != null) {
System.out.println(current.data);
current = current.next;
}
}
If you count the number of nodes in the linked list, you can also write this with a counter-controlled loop.
public void traverse() {
Node<T> current = head;
for (int count = 0; count < numElements; count++) {
System.out.println(current.data);
current = current.next;
}
}