Build a Linked List
- Trace the basic operations of a (singly) linked-list implementation.
Consider the following implementation of Node
(with package-private visibility modifier):
class Node {
int data;
Node next;
Node(int data) {
this.data = data;
this.next = null; // we can eliminate this line; it happens by default
}
}
Exercise Draw a schematic representation of a linked list pointed to by the head
variable after the following code snippet has been executed.
Node head = new Node(7);
head.next = new Node(5);
head.next.next = new Node(10);
Node node = new Node(9);
node.next = head;
head = node;
Solution
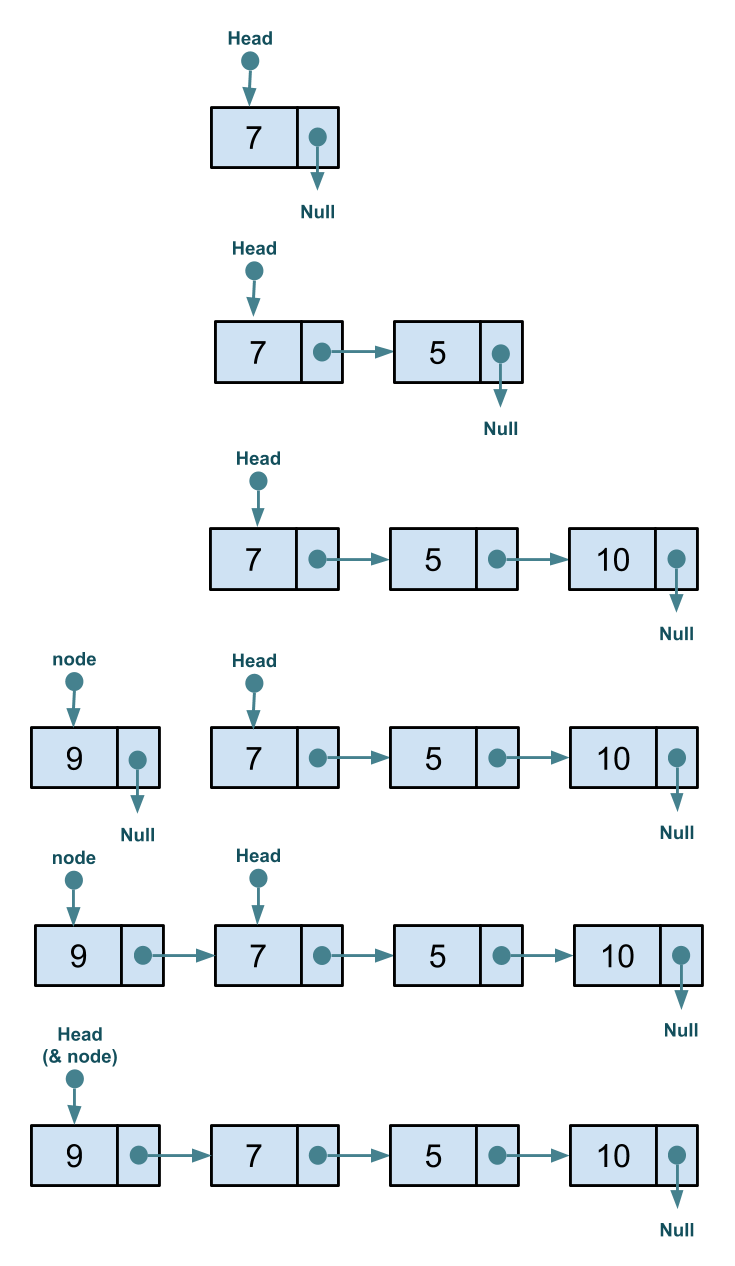