Append Operation
- Trace the basic operations of a (singly) linked-list implementation.
- Understand the basic operations of a (singly) linked list well enough to implement them.
Suppose we have the following linked list and we want to append (add to the end) a new node.
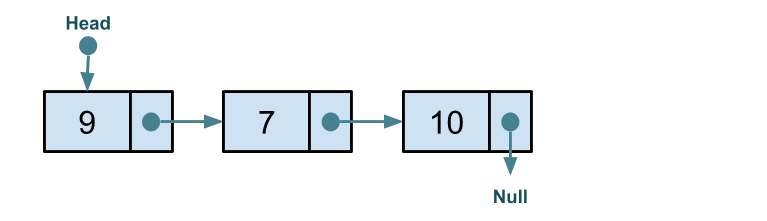
Exercise Complete the implementation of the addLast
method that creates a node and add it to the back of the list.
public void addLast(T t) {
// TODO Implement me!
}
Hint: Use the following visualization as guidance.
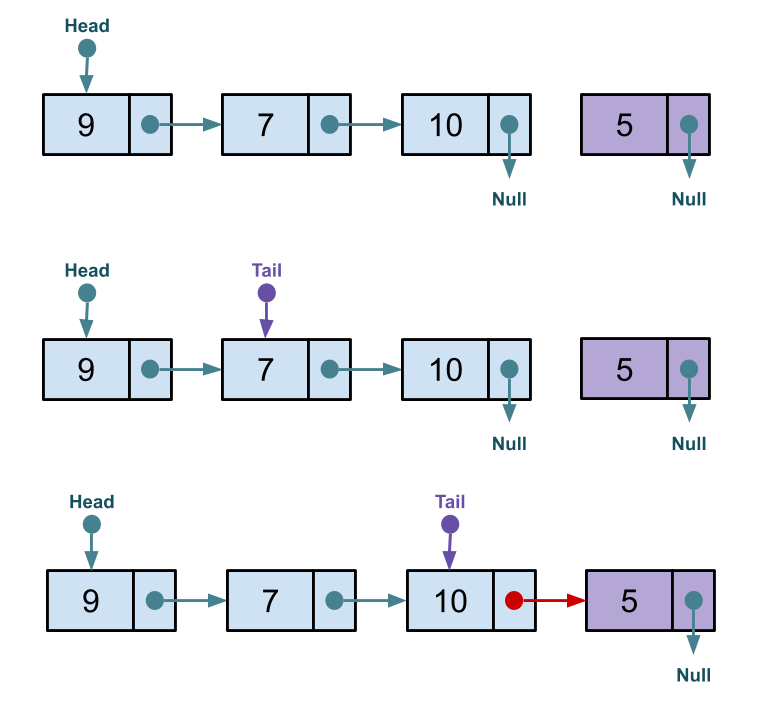
Solution
public void addLast(T t) {
Node<T> tail = head;
while (tail.next != null) {
tail = tail.next;
}
tail.next = new Node<T>(t);
}
If you count the number of nodes in the linked list, you can also write this with a counter-controlled loop. In that case, the find
helper method from when we implemented get
can be used here to go to the last element.
Caution: the implementation above fails to account for an edge case!